Run Targets: JUnit: attempt to run 'all in package' run configuration fails with SSH target: Editor. Diff and Merge: Bug: IDEA-257651: Diff view detects big diff but change was only small: Lang. Markdown: Bug: IDEA-258796: Unnecessary backslash escape in triple-backticked bash block: Lang. XPath and XSLT: Bug: IDEA-207408: XSLT debugger broken. Open your project in PhpStorm and open the DataSource window: Click on View - Tool Windows - Database; Click on the Database sidebar, click on new - Datasource - MySQL The configuration window will appear; Step 2: configure the ssh tunnel. Open your terminal. Cd /path/to/your/project.
When you work with remote servers, a common and recommended security measure is to use SSH key pairs for authentication.
Our friends at PyCharm have prepared a great blog post on the topic. It explains how to generate an SSH key pair, store the passphrase for the private key in the credential helper, and, in the end, how to connect to a remote Python interpreter over SSH using the generated key pair for authentication.

Before proceeding, make sure that you have a remote machine at hand to which you can connect over SSH using the generated key pair. Also, make sure to add your key to the credential helper application (SSH agent, Pageant, or a compatible tool). If you don’t have a spare remote machine, you can use the example Vagrant box provided as part of the above tutorial.
When ready, jump right into the PhpStorm specifics.
In PhpStorm, we can make use of SSH keys and credential helper applications in two areas: when configuring remote deployment servers and remote PHP interpreters. Both scenarios involve the same setup technique, and we can actually reuse an existing remote deployment server configuration for setting up an interpreter.
Setting up a remote deployment server
To connect to a remote deployment server over SSH, we need to create a new server access configuration of the SFTP type.
Navigate to Settings / Preferences | Build, Execution, Deployment | Deployment. Then, click + to add a new SFTP server:
Next, provide the server parameters:
In this tutorial, we’ll use the values that will allow us to connect to the example Vagrant box:
- SFTP host: localhost
- Port: 2222 (the default port that Vagrant exposes for SSH).
- User name: example
- Auth type: Authentication agent (ssh-agent or Pageant)
By setting authentication method to Authentication agent, you’re instructing PhpStorm to use the credentials stored in the credential helper application (SSH agent, Pageant, or a compatible tool).
Once the parameters are set, test your connection, apply the changes, and you’re good to go!
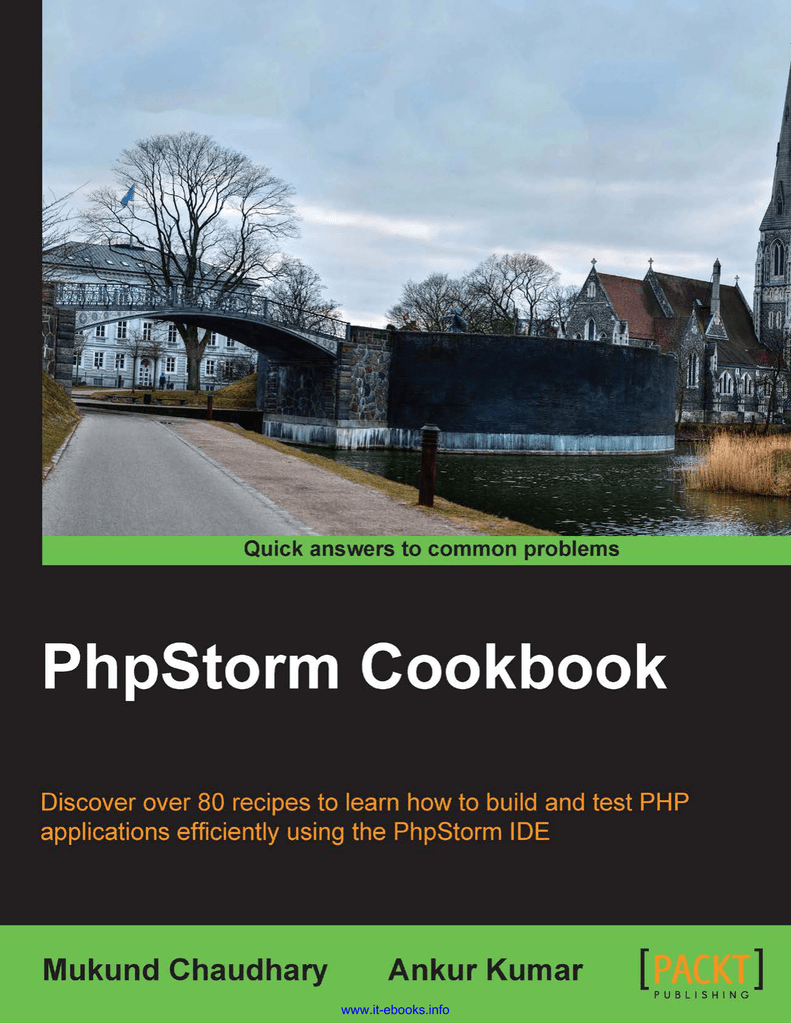
The remote server that we have configured will probably handle our PHP code as well. We can reuse its configuration for setting up a remote PHP interpreter. First, let’s ensure that PHP is installed on the remote server. Select Tools | Start SSH Session… in the main menu and select the remote host in the pop-up window:
Since your SSH keys are managed by the credential helper, the SSH session should instantly start in the built-in PhpStorm terminal without requiring you to type anything. To find out whether PHP is installed, run the php -v
command:
The command is not found, which means that PHP is not installed. We can easily fix this by running the following command:
sudo apt-get install php
Note that this command requires elevated privileges, i.e. entering your password. In our Vagrant box, the password for the example user is set to hunter2. Type it in when prompted, and wait a short while until PHP is installed. To be absolutely sure, you can run the php -v
command once more:
Setting up a remote PHP interpreter
PHP is now installed on a remote server, and instructing PhpStorm to use it could not be simpler. Navigate to Settings / Preferences | Languages & Frameworks | PHP and click the ellipsis (…) button next to the CLI Interpreter field:
In the opened CLI Interpreters window, click + and select the From Docker, Vagrant, VM, Remote… interpreter type:
PhpStorm will detect a remote deployment server configuration that we created earlier and will suggest reusing it as a configuration for the remote interpreter:
Click OK, apply your changes, and you are done! You can now upload, download, and manage remote files directly in PhpStorm, as well as execute your PHP code remotely.
As a side note, if you need to set up only a remote PHP interpreter, without reusing an existing deployment configuration, choose the SSH Credentials option in the above dialog box. Provide the server parameters and select the Authentication agent (ssh-agent or Pageant) authentication type – just as you would for configuring a remote deployment server.
JetBrains PhpStorm Team
The Drive to Develop
What I wanted to achieve

As I am using DDEV for most of my projects as simple docker environment for web development and PHPStorm as IDE I wanted to be able to run test from PHPStorm - not only as a script, but fully integrated with coverage and test debugging. Basically, what I wanted to achieve is what you see on the screenshot:
PHPStorm has a pretty good docker integration if we are talking about docker run
or docker-compose run
. That means, with the PHPStorm docker integration, you can use a docker image to run your tests, however you cannot connect to an existing, running docker container and use that to run your scripts. With DDEV, that is what we would need: We have DDEV running and now want PHPStorm to execute our tests in the DDEV environment.
Solution
While we cannot use PHPStorms docker integration, what we can use is the SSH integration. PHPStorm also offers the option to add a remote interpreter over SSH. This gave me a starting point, so what we need to do to get it running is:
- Install an SSH server on our DDEV web container
- Make the SSH server accessible from the host
- Allow authentication with our private key
- Add PHP over SSH as Remote Interpreter in PHPStorm
- Add PHPUnit by Remote Interpreter
Let's take a look - step by step:
Install an SSH Server in DDEV
First of all, I added the ssh
package to the DDEV web image by adding the following line in DDEV's config.yaml
:
Next I added a post-start hook to start the SSH server when starting DDEV in config.yaml
:
When starting DDEV for the first time after adding this, the container image will be rebuilt with the SSH package and the SSH server will be started.
Make the SSH server accessible from the host
To allow connecting to the DDEV SSH server via the host from all platforms we need to forward the SSH port to the host. To do that, I added a file docker-compose.overwrite.yaml
in the .DDEV
folder with the following content:
This config makes our DDEV SSH server accessible via port 9922 from the host (can be used with our DDEV site name as host - in my case: site-shop.DDEV.site:9922
).
Note
When using Linux based host systems (not Mac, not Windows, not WSL) you can directly access the SSH server via the container IP.
On systems using a virtualization layer in between (Windows/Mac) the IPs cannot be directly accessed - the port solution works around that limitation and allows us to use a more speaking name, too :)
Allow Authentication with our private key
Phpstorm Sftp
As we mostly have an SSH agent running for all the things we do anyway, we should access our DDEV SSH server with that key, too, instead of using password based authentication. To achieve that, we need to add our public key to the server's authorized_keys
file. DDEV has a feature called homeadditions
which allows us to add files to our config that will be mounted in the home dir of our DDEV instance. That's perfect. Add the following structure in the folder .ddev/homeadditions
:
Phpstorm Split Screen
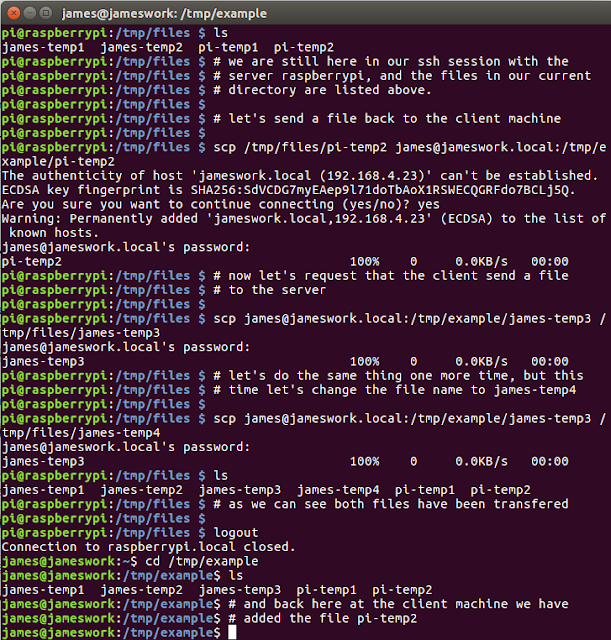
Then add your public key to the authorized_keys
file.
Tip
If you are using DDEV > 1.15 you can add your authorized_keys
file to your global home additions. See https://ddev.readthedocs.io/en/stable/users/extend/in-container-configuration/
Otherwise, add the authorized_keys file to the .gitignore - especially if you are working on your projects with multiple people. That way you all can have your keys set up locally.
To ensure the file rights on the key are corrected, I extended my post-start hooks with a chmod
command (replace the username with your username):

After restarting DDEV you should now be able to connect to your DDEV SSH server with your key. Try it:
Phpstorm Scratch File
Add PHP over SSH as Remote Interpreter in PHPStorm
Now that our SSH server is running, we need to configure our PHPStorm to run PHP via SSH.
- Open PHPStorm
- Go to Settings > Languages & Frameworks > PHP
- Click the tripe dot
...
next to CLI interpreters - Click the
+
sign and choose theFrom Docker, Vagrant, Vm, WSL, Remote
option - Choose
SSH
and configure the SSH connection - Save all settings
Tip
With this config it's already possible to run all PHP script related things in PHPStorm in the DDEV environment.
Add PHPUnit by Remote Interpreter
As a last step to get our PHPUnit tests running via SSH we need to add a PHPUnit configuration - in PHPStorm:
- Go to Settings > Languages & Frameworks > PHP > Testing Frameworks
- Choose the SSH PHP interpreter we created in the previous step as CLI Interpreter
- Set up path mappings to allow PHPStorm to find the files
- Load phpunit via composer autoloader - the file dialog should already display the remote file system
- Add a configuration file if necessary
All done, you are ready to run tests directly in PHPStorm.
Run Tests
After completing the setup you can now run tests in PHPStorm. If everything is set up correctly you can for example:
- Right click a
phpunit.xml
config file and chooseRun
- Open a test file and run the file or a single test by clicking the green arrow icon
Phpstorm Split Window
Debugging Tests
If you want to debug tests or run the tests with coverage, you need to enable xdebug first:
after that, you can use the bug icon in PHPStorm to run the tests with a debugger available.
Bonus: Run composer scripts via ddev
In some projects we are making heavy use of composer scripts as shortcuts for running tests, cgl fixer, PHPStan etc. With the setup we have now, it's easy to also run these scripts via our ddev interpreter. The configuration is similar to the PHPUnit configuration:
- Go to
Settings > Languages & Frameworks > PHP > Composer
- Choose the remote SSH Cli Interpreter
- Set up the path mappings
- Add
composer
as composer executable
As a result you will now get the 'little green arrow' in the composer.json
file, allowing you to run the scripts in PHPStorm on your DDEV environment with a single click.
Feedback welcome
That's it for now, if you have any feedback, don't hesitate to contact me via Twitter (@sasunegomo) or TYPO3 Slack (@susi).
